Właściwości i Metody klasy String
Klasa System.String
w języku C# posiada dwie
główne właściwości oraz wiele Metod.
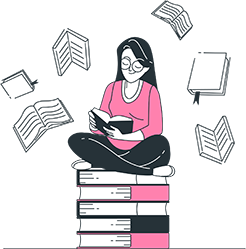
Właściwość Length
string str = "Hello World!";
int length = str.Length; // length zawiera wartość 12
12
Właściwość Chars[]
string str = "Hello World!";
char firstChar = str[0]; // firstChar zawiera wartość 'H'
H
Metoda Concat
string str1 = "Hello";
string str2 = " World";
string str3 = string.Concat(str1, str2); // str3 zawiera wartość "Hello World"
Hello World
Metoda Compare
string str1 = "Hello";
string str2 = "World";
int result = string.Compare(str1, str2); // result zawiera wartość -15
-15
Metoda Contains
string str = "Hello World";
bool result = str.Contains("World"); // result zawiera wartość true
true
Metoda Substring
string str = "Hello World";
string result = str.Substring(6); // result zawiera wartość "World"
World
Metoda ToLower
string str = "Hello World";
string result = str.ToLower(); // result zawiera wartość "hello world"
hello world
Metoda Replace
string str = "Hello World";
string result = str.Replace("World", "Universe"); // result zawiera wartość "Hello Universe"
Hello Universe
Metoda Split
string str = "Hello World";
string[] result = str.Split(' '); // result zawiera tablicę zawierającą "Hello" i "World"
Hello
World
World
Metoda Trim
string str = " Hello World ";
string result = str.Trim();
Metoda Remove
string str = "Hello World";
string result = str.Remove(6, 5); // result zawiera wartość "Hello"
Hello
Metoda IndexOf
string str = "Hello World";
int result = str.IndexOf("World"); // result zawiera wartość 6
6
Metoda LastIndexOf
string str = "Hello World, World";
int result = str.LastIndexOf("World"); // result zawiera wartość 13
13
Metoda Insert
string str = "Hello";
string result = str.Insert(5, " World"); // result zawiera wartość "Hello World"
Hello World
Metoda Join
string[] strArray = { "Hello", "World" };
string result = string.Join(" ", strArray); // result zawiera wartość "Hello World"
Hello World
Metoda PadLeft
string str = "Hello";
string result = str.PadLeft(10, '*'); // result zawiera wartość "****Hello"
****Hello
Metoda PadRight
string str = "Hello";
string result = str.PadRight(10, '*'); // result zawiera wartość "Hello****"
Hello****